Object Oriented Programming With Real world Example
Fundamentally, the purpose of coding is to address real-world problems. In Object-Oriented Programming (OOP), the logic is structured around the concept of objects, incorporating key principles such as abstraction, encapsulation, inheritance, and polymorphism.
Consider various real-world entities like cars, bikes, ATMs, and coffee machines, each with different brands and names. In OOP, these entities are referred to as objects.
The logic for objects is implemented through classes. For instance, a phone class may include functionalities like making calls, Bluetooth connectivity, and taking photos. Each of these functionalities is organized into classes. When creating classes, it's crucial to consider the SOLID principles, ensuring a robust and maintainable code structure.
Abstraction
Before delving into abstraction in Object-Oriented Programming (OOP), let's first understand its meaning in English. Abstraction involves revealing only relevant data and concealing extraneous details—this stands as a cornerstone in OOP. Typically, abstraction is achieved through interfaces rather than abstract classes.
Interface:
Abstraction can be achieved through classes or interfaces. Abstract classes may lack implemented methods, whereas abstract classes may include them. Drawing a parallel, consider a remote acting as an interface between a TV and its user.
Choosing Between Interface and Abstract Classes:
The decision between interfaces and abstract classes depends on your requirements. If you necessitate multiple inheritances and a clear blueprint focusing solely on design (without implementation), interfaces are the preferred choice. On the other hand, if you seek a blend of design planning and pre-implementation without multiple inheritances, abstract classes suit your needs.
Real-World Examples:
- Making a call serves as an illustration of abstraction, where the process involves concatenating numbers and displaying them on the screen. The intricacies of connecting with another number remain concealed.
- When sending data via Bluetooth, the abstraction is evident as we do not delve into the details of how the connection is established with other phones or devices.
Encapsulation
Abstraction and Encapsulation operate synergistically, as abstraction must showcase details at a level accessible only through encapsulation.
Real-time Application of Encapsulation:
In real-world scenarios, we employ Encapsulation for security purposes.
Examples:
Enabling Bluetooth illustrates encapsulation, where connection to other phones is possible, but accessing calls or sending SMS from that phone remains hidden within the encapsulation. Some abstraction elements contribute to this.
When connecting with phone 'x' and 'x' connects with phone 'y', the encapsulation ensures restricted access. In this scenario, I lack the authorization to connect with phone 'y' through phone 'x'. Such concealment is managed by encapsulation.
Access Specifiers:
Access specifiers like public, private, protected, and internal play a pivotal role in handling these encapsulation and abstraction scenarios.
Polymorphism
A straightforward illustration of this concept is when we activate a phone with a single button, we can also power it off using the same button simultaneously. This simplicity highlights the principle of toggling the phone's state seamlessly through a singular control.
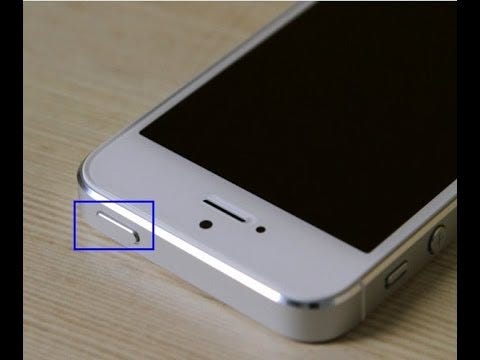
Example
We can send normal text messages and we can send video messages also.
Here we need to change the mode.
Inheritance
In Object-Oriented Programming (OOP), the act of creating a new class (sub-class) derived from a base or superclass is referred to as inheritance. Through inheritance, all the attributes and behaviors from the base/superclass are inherited by the subclass. This mechanism facilitates the reuse of code and allows for the extension and specialization of functionalities in a structured manner.
There are mainly 4 types of inheritance:
- Single level inheritance
- Multi-level inheritance
- Hierarchical inheritance
- Hybrid inheritance
- Multiple inheritance
Single level inheritance
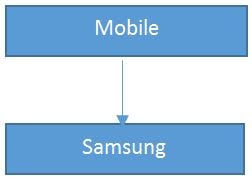
Here some features of mobile are used in the Samsung brand.
Multi-level inheritance
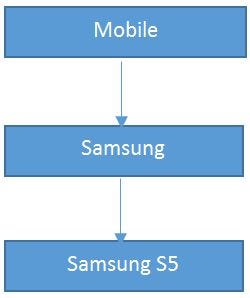
S5 has more features than Samsung J1, so they use new features with old features.
Hierarchical inheritance
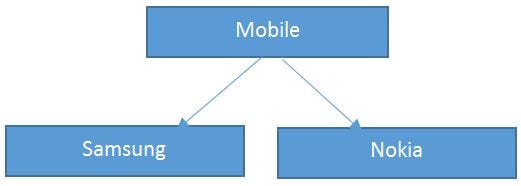
Some of the features may be used in two types of brands.
Hybrid inheritance
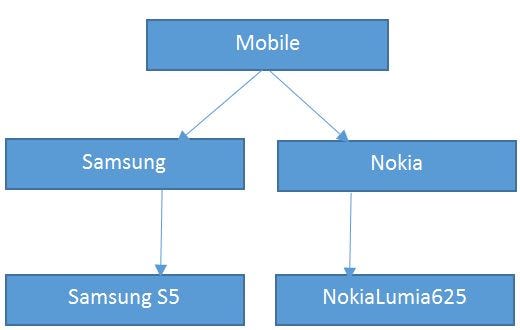
Some of the features may be used in two types of brands, and those brands may publish new brands with new features.
Multiple inheritance
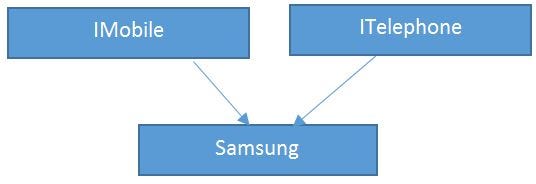
Reference:
Comments
Post a Comment